1.API
1.1Introduction
As of version 2.0, TeamBooking implements a REST API to make the interaction with its data easier for developers, or customizations of the data flow.
Important: this chapter is intended for developers. If you don't know what an API is, you can skip the reading.
Note: the API is still at early stage, so it could have some limits. Feel free to contact our support for any suggestion.
1.2Auth tokens
In order to use the API, a client must provide an authorization token to identify itself.
Authorization tokens can be generated inside the Core Settings
of TeamBooking's dashboard.
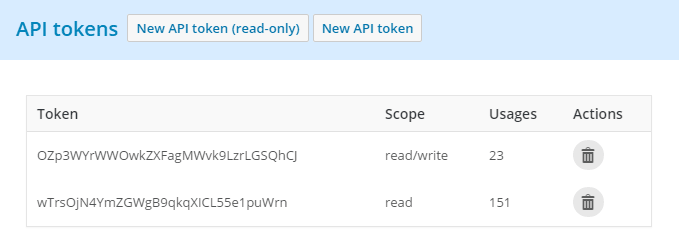
You can create a read-only token, or a token allowed to authorize writing data operations (e.g. edit, delete and so on).
A token can't be modified, but can be revoked (deleted).
1.3Requests
Here's the list of currently available requests:
-
Reservations list
GET
-
Get reservation
GET
-
Services list
GET
-
Get slots
GET
-
Edit reservation
POST
-
Do reservation
POST
-
Cancel reservation
POST
-
Delete reservation
POST
1.3.1Reservations list (GET)
Returns the reservation entries.
GET YOUR-DOMAIN/wp-admin/admin-ajax.php?action=teambooking_rest_api
Parameter | Required | Value | Description |
---|---|---|---|
operation
|
yes |
get_reservations
|
Must be provided with this value, to trigger the operation. |
auth_token
|
yes | string | The authorization token to perform the operation. |
coworker
|
no | int | Coworker's ID. If specified, the response will contain only the reservations that belong to the coworker. |
service_id
|
no | string | Service's ID. If specified, the response will contain only the reservations for that service. |
show_past
|
no | bool |
If TRUE , the expired reservations will be included in the response. Default is TRUE .
|
If successful, this method returns a response body with the following structure:
{ "header": "200 OK", "code": 200, "response": [ reservation Resource ] }
1.3.2Get reservation (GET)
Returns the specified reservation entry.
GET YOUR-DOMAIN/wp-admin/admin-ajax.php?action=teambooking_rest_api
Parameter | Required | Value | Description |
---|---|---|---|
operation
|
yes |
get_reservation
|
Must be provided with this value, to trigger the operation. |
auth_token
|
yes | string | The authorization token to perform the operation. |
id
|
yes | int | The reservation's ID. |
If successful, this method returns a response body with the following structure:
{ "header": "200 OK", "code": 200, "response": reservation Resource }
1.3.3Services list (GET)
Returns the services list.
GET YOUR-DOMAIN/wp-admin/admin-ajax.php?action=teambooking_rest_api
Parameter | Required | Value | Description |
---|---|---|---|
operation
|
yes |
get_services
|
Must be provided with this value, to trigger the operation. |
auth_token
|
yes | string | The authorization token to perform the operation. |
If successful, this method returns a response body with the following structure:
{ "header": "200 OK", "code": 200, "response": [ service Resource ] }
1.3.4Get slots (GET)
Returns the free slots entries.
GET YOUR-DOMAIN/wp-admin/admin-ajax.php?action=teambooking_rest_api
Parameter | Required | Value | Description |
---|---|---|---|
operation
|
yes |
get_slots
|
Must be provided with this value, to trigger the operation. |
auth_token
|
yes | string | The authorization token to perform the operation. |
service_id
|
yes | int | The service's ID. |
If successful, this method returns a response body with the following structure:
{ "header": "200 OK", "code": 200, "response": [ slot Resource ] }
1.3.5Edit reservation (POST)
Edit a reservation entry.
POST YOUR-DOMAIN/wp-admin/admin-ajax.php
Parameter | Required | Value | Description |
---|---|---|---|
action
|
yes |
teambooking_rest_api
|
Must be provided with this value, to trigger the APIs. |
operation
|
yes |
edit_reservation
|
Must be provided with this value, to trigger the operation. |
auth_token
|
yes | string | The authorization token to perform the operation. NOTE: it must have been issued for write operations. |
reservation
|
yes | reservation Resource |
The edited reservation resource. Please note that read_only properties will not be changed, even if you provide changes to them.
|
If successful, this method returns a response body with the following structure:
{ "header": "200 OK", "code": 200 }
1.3.6Do reservation (POST)
Make a reservation.
POST YOUR-DOMAIN/wp-admin/admin-ajax.php
Parameter | Required | Value | Description |
---|---|---|---|
action
|
yes |
teambooking_rest_api
|
Must be provided with this value, to trigger the APIs. |
operation
|
yes |
do_reservation
|
Must be provided with this value, to trigger the operation. |
auth_token
|
yes | string | The authorization token to perform the operation. NOTE: it must have been issued for write operations. |
reservation
|
yes | reservation Resource | The reservation resource. |
slot
|
yes | slot Resource | The slot resource (one of the slot resources you get from the get_slots call). |
If successful, this method returns a response body with the following structure:
{ "header": "200 OK", "code": 200, "response": { "response":1, "state":"confirmed", "reservation_id":166, "redirect":"http://www.google.com" } }
The response contains some useful data: the status of the reservation, the reservation's ID and (if any) the URL where the customer is supposed to be redirected after the reservation.
1.3.7Cancel reservation (POST)
Cancel a reservation.
POST YOUR-DOMAIN/wp-admin/admin-ajax.php
Parameter | Required | Value | Description |
---|---|---|---|
action
|
yes |
teambooking_rest_api
|
Must be provided with this value, to trigger the APIs. |
operation
|
yes |
cancel_reservation
|
Must be provided with this value, to trigger the operation. |
auth_token
|
yes | string | The authorization token to perform the operation. NOTE: it must have been issued for write operations. |
id
|
yes | integer | The reservation's ID |
If successful, this method returns a response body with the following structure:
{ "header": "200 OK", "code": 200 }
Sometimes, it could be present additional information in the response:
{ "header": "200 OK", "code": 200, "response" : string }
1.3.8Delete reservation (POST)
Delete a reservation entry.
POST YOUR-DOMAIN/wp-admin/admin-ajax.php
Parameter | Required | Value | Description |
---|---|---|---|
action
|
yes |
teambooking_rest_api
|
Must be provided with this value, to trigger the APIs. |
operation
|
yes |
delete_reservation
|
Must be provided with this value, to trigger the operation. |
auth_token
|
yes | string | The authorization token to perform the operation. NOTE: it must have been issued for write operations. |
id
|
yes | integer | The reservation's ID |
If successful, this method returns a response body with the following structure:
{ "header": "200 OK", "code": 200 }
1.4Resources
A resource is a data object with properties and values. Here's a list of current TeamBooking API resources.
1.4.1Reservation resource
The reservation resource has the following structure:
{ "type" : (read-only) "reservation", "id" : (read-only) integer, "coworkerID" : (read-only) integer, "customerUserID" : integer, // "0" if not registered "customerTimezone" : string, "service" : { "id" : string, "location" : string }, "datetime" : { "start" : integer, // Unix time in seconds "end" : integer // Unix time in seconds }, "payment" : { "amount" : integer, "isPaid" : boolean, "currency" : integer, "gateway" : string }, "status" : string, // "confirmed", "pending", "waiting_approval", "done" "tickets" : integer, "creationDateTime" : (read-only) integer, // Unix time in seconds "reminderSent" : boolean, "referer" : { "postID" : integer, "postTitle" : string }, 'formFields' : [ form_field Resource ] }
1.4.2Service resource
The service resource has the following structure:
{ "type" : (read-only) "service", "id" : (read-only) string, "name" : string, "class" : (read-only) string, // "event", "appointment", "unscheduled" "color" : string, "description" : string, "isActive" : boolean, "notificationEmailAdmin" : { "send" : boolean, "address" : string, "subject" : string, "body" : string }, "confirmationEmail" : { "send" : boolean, "subject" : string, "body" : string }, "reminderEmail" : { "send" : boolean, "subject" : string, "body" : string }, "approval" : { "requireFrom" : string, // "admin", "coworker", "none" "keepFreeUntilApproval" : boolean }, "cancellationByCustomer" : { "allow" : boolean, "timespan" : integer //seconds, relative to the reservation's start time }, "payments" : { "price" : integer, "required" : string, // "immediately", "later", "discretional" }, "loggedOnly" : boolean, "maxTotalTicketsPerSlot" : integer, "maxUserTicketsPerSlot" : integer, "maxReservationsPerLoggedUser" : integer, "location" : { "setting" : string, // "none", "inherited", "fixed" "address" : string }, "redirectURL" : string, "assignment" : { "rule" : string, // "equal", "direct", "random", "none" "directCoworkerID" : integer } }
1.4.3Slot resource
The slot resource has the following structure:
{ "type" : (read-only) "slot", "serviceID" : (read-only) string, "serviceName" : (read-only) string, "serviceDescription" : (read-only) string, "location" : string, "coworkerID" : integer, "isSoldout" : boolean, "isAllday" : boolean, "tickets" : integer, "start" : string, "end" : string, "gcalEvent" : string, "gcalParentEvent" : string, "gcalID" : string, }
1.4.4Form field resource
The form_field resource has the following structure:
{ "type" : (read-only) "form_field", "name" : string, "value" : string, "priceIncrement" : integer }